Stencil.js is a compiler for WebComponents. It lets you write Web Components in TypeScript and jsx. If you are new to Stencil.js please take a look at their site https://stenciljs.com for a brief introduction.
You can download the starter project by simply cloning it so that you can skip the configuration part which may take ages.
git clone https://github.com/ionic-team/stencil-app-starter my-pwa
You can then install the dependencies by using the following command inside the my-pwa
folder
npm install
Hello World
Let’s take a look at a small Hello World Example to get ourselves familiar with the starter template. Create a file hello-world.tsx
inside the my-pwa/src/components/hello-world/
folder.
// filename: hello-world.tsx
import { Component } from '@stencil/core';
@Component({
tag: 'hello-world',
styleUrl: 'hello-world.css'
})
export class HelloWorld {
render() {
return (
<h1>Hello World</h1>
);
}
}
The part @Component
is a decorator which gives you information about the html tags used and the style sheet used. As you can see that we are trying to use a css file called hello-world.css
for this stylesheet, let’s first create this file inside the same hello-world
folder.
hello-world h1 {
color: red;
}
Now, in order to load this component on to the page, we need to use it first. Let’s add the component <hello-world>
in the <app-home>
component.
In file app-home.tsx
inside the ` my-pwa/src/components/app-home/` folder we will simply add the hello-world
component.
Before:
<p>
Welcome to the Stencil App Starter.
You can use this starter to build entire apps all with
web components using Stencil!
Check out our docs on
<a href='https://stenciljs.com'>stenciljs.com</a>
to get started.
</p>
<stencil-route-link url='/profile/stencil'>
<button>
Profile page
After:
<p>
Welcome to the Stencil App Starter.
You can use this starter to build entire apps all with
web components using Stencil!
Check out our docs on
<a href='https://stenciljs.com'>stenciljs.com</a>
to get started.
</p>
<hello-world></hello-world>
<stencil-route-link url='/profile/stencil'>
<button>
Profile page
Once this is done, we can now use the npm command to start the server and launch the page on to the browser with our hello world component.
npm run dev
The output looks like this
Upcoming in Part 02
This blog just gives you a basic introduction to Stencil.js and how we can use the startup template to have our own hello-world
webcomponent in a PWA. In the next blog in this series, we will be looking at Mail Client App by Jakub Antalik (refer screenshot below) and how we can create it in our demo.
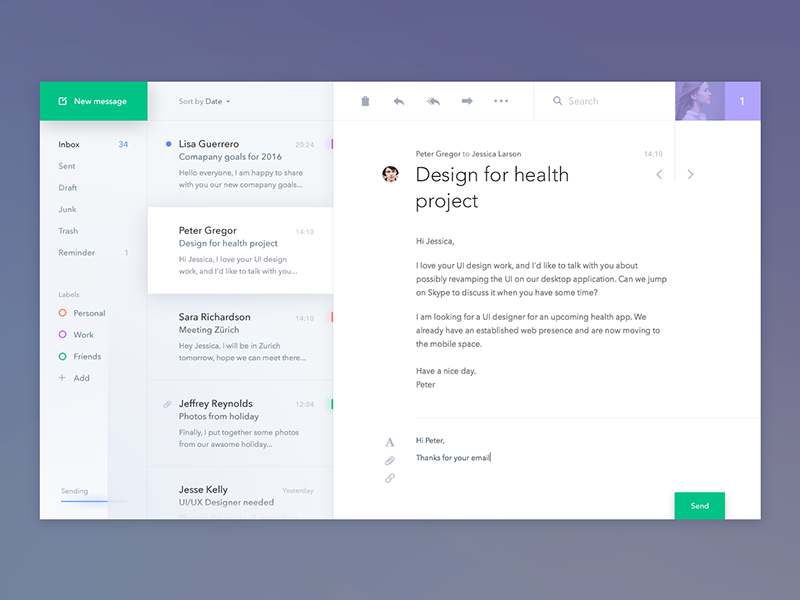
If you want to take a look at the source code for this my-pwa
folder, feel free to refer this link https://github.com/prateekjadhwani/my-pwa-01 .